14_集合,
分享于 点击 25841 次 点评:217
14_集合,
集合 为什么会出现集合框架 [1] 之前的数组作为容器时,不能自动拓容 [2] 数值在进行添加和删除操作时,需要开发者自己实现添加和删除。 Collection接口 Collection基础API Java集合框架提供了一套性能优良、使用方便的接口和类,它们位于java.util包中。 Collection表示集合的根接口,可以看成一个容器,存储了很多对象,这些对象称为Collection元素。Collection要求元素必须是引用数据类型。 Collection 根据其元素的特征可以分为 List接口->元素有序、可重复 Set 接口-> 元素无序、不可重复![]() |
public class Test01 { public static void main(String[] args) { /** * 增:add/addAll * 删:clear/remove/removeAll/retainAll * 改: * 查:contains/constainsAll/size/isEmpty * 其他:equals * 遍历:iterator() */ Collection col1 = new ArrayList(); col1.add("apple"); // Object obj = "apple" 多态 col1.add("banana"); // Integer i = 1; 装箱 // 注意:Collection可以添加任意类型的数据,最好添加统一类型的数据方便未来统一访问。 Collection col2= new ArrayList(); col2.add("apple"); col2.add("banana"); // col1.addAll(col2); //System.out.println(col1); //col1.clear(); // 清空集合 //col1.remove("apple"); // 移除指定元素 //col1.removeAll(col2); // 移除指定集合中的多个元素 //col1.retainAll(col2); // 保留指定集合col2中的元素,其他删除 //System.out.println(col1); //col1.remove(1); // [apple, banana, 2] //System.out.println(col1.contains("apple")); //System.out.println(col1.containsAll(col2)); //System.out.println(col1.size()); // 返回元素个数 //System.out.println(col1.isEmpty()); boolean r = col1.equals(col2); System.out.println(r); } } |
public static void main(String[] args) { Collection col1 = new ArrayList(); col1.add("apple"); col1.add("coco"); col1.add("banana"); // 遍历集合中的元素 /* * Object 表示迭代元素类型 * item 表示迭代变量 * */ // 快速遍历 for (Object item : col1) { System.out.println(item); } // 迭代器遍历 Iterator it1 = col1.iterator(); // 返回集合的迭代器 while(it1.hasNext()) { String item = (String)it1.next(); System.out.println(item); } // 写法(更节省内存) for(Iterator it2 = col1.iterator();it2.hasNext();) { String item = (String)it2.next(); System.out.println(item); } } |
public static void main(String[] args) { /** * 增:add(index,e)/addAll(index,c)/add(e)/addAll(c)/ * 删:remove(index)/clear()/remove(e)/removeAll(c)/retainAll(c) * 改:set(index,e); * 查:get(index)/indexOf(e)/lastIndexOf(e)/contains/constainsAll/size/isEmpty * 其他:equals * 遍历:iterator() / listIterator() */ List list1 = new ArrayList(); // 【1】添加 // 追加 list1.add("apple"); list1.add("banana"); // 在指定index位置添加元素coco list1.add(0,"coco"); List list2 = new ArrayList(); list2.add(1); list2.add(2); list1.addAll(0, list2); System.out.println(list1); // 【2】移除 //list1.remove(0); //System.out.println(list1); // 【3】修改 list1.set(0, 100); System.out.println(list1); // 【4】查看元素 可能产生IndexOutOfBoundsException // System.out.println(list1.get(6)); System.out.println(list1.indexOf(200)); // list1.add(2); // System.out.println(list1.lastIndexOf(2)); } |
public static void main(String[] args) { // List 集合的遍历 List list = new ArrayList(); list.add("apple"); list.add("banana"); list.add("coco"); // 【1】 for 循环 for(int i=0;i<list.size();i++) { String item = (String) list.get(i); System.out.println(item); } // 【2】 快速遍历 for(Object item:list) { System.out.println(item); } // 【3】iterator Iterator it1 = list.iterator(); while(it1.hasNext()) { System.out.println(it1.next()); } // 【4】listIterator 获取列表的迭代器 ListIterator it2 = list.listIterator(); // 正向遍历(A) while(it2.hasNext()) { System.out.println(it2.next()); } // 逆向遍历(B) while(it2.hasPrevious()) { System.out.println(it2.previous()); } // 【5】从指定位置开始迭代(C) System.out.println("--listIterator(index)--"); ListIterator it3 = list.listIterator(1); while(it3.hasNext()) { System.out.println(it3.next()); } } |
![]() |
![]() |
![]() |
![]() |
public class Test01 { public static void main(String[] args) { List list1 = new LinkedList(); // 【1】添加 list1.add("apple"); list1.add("banana"); list1.add("coco"); System.out.println(list1); // 【2】删除 list1.remove("apple"); // 【3】修改 list1.set(0, "banana x"); System.out.println(list1); // 【4】查看 System.out.println(list1.get(0)); } } |
![]() |
public class Test02 { public static void main(String[] args) { // 以栈形式访问 LinkedList stack1 = new LinkedList(); // 入栈 stack1.push("apple"); stack1.push("banana"); // 出栈 System.out.println(stack1.pop()); System.out.println(stack1.pop()); // 如果栈中没有元素,抛出NoSuchElementException System.out.println(stack1.pop()); } } |
![]() |
public static void main(String[] args) { // 以队列形式访问 LinkedList queue = new LinkedList(); // 入队 /* 头(出口)<----------尾(入口) --------------------- apple banana coco --------------------- */ //queue.add("apple"); //queue.add("banana"); //queue.add("coco"); //System.out.println(queue); // 出队 //queue.remove(); //queue.remove(); //queue.remove(); // 抛出NoSuchElementException //queue.remove(); //System.out.println(queue); // 检测元素:获取但不移除此列表的头(第一个元素) //System.out.println(queue.element()); //System.out.println(queue.element()); /*queue.offer("apple"); queue.offer("banana"); queue.offer("coco");*/ System.out.println(queue); //queue.poll(); //System.out.println(queue); //queue.poll(); //queue.poll(); // 如果队列为空,返回null //String item = (String)queue.poll(); //System.out.println(item); System.out.println(queue.peek()); } |
![]() |
public static void main(String[] args) { // 以双向队列形式访问 LinkedList queue = new LinkedList(); /* * 头 尾 <------------------- --------------------- apple banana coco --------------------- -------------------> */ queue.addFirst("apple"); queue.addLast("banana"); queue.addLast("coco"); //queue.removeFirst(); //queue.removeLast(); //queue.removeLast(); // NoSuchElementException //queue.removeLast(); //System.out.println(queue); System.out.println(queue.getFirst()); System.out.println(queue.getLast()); } |
public static void main(String[] args) { ArrayList<Integer> list = new ArrayList<Integer>(); System.out.println(list instanceof ArrayList); System.out.println(list instanceof ArrayList<?>); System.out.println(list instanceof ArrayList<Integer>); //error } |
Cannot perform instanceof check against parameterized type ArrayList<Integer>. Use the form ArrayList<?> instead since further generic type information will be erased at runtime |
public class Student<T> { private T t; public T getT() { return t; } public void setT(T t) { this.t = t; } } |
public class Student{ /* public void add(int a,int b) { System.out.println(a+b); } public void add(float a,float b) { System.out.println(a+b); } public void add(char a,char b) { System.out.println(a+b); } public void add(String a,String b) { System.out.println(a+b); } */ public <T> void add(T a,T b) { System.out.println(a.toString()+b.toString()); } } |
// 方法的可变参数 /*public void add(int...args) { // 方法的可变参数在方法调用时,以args数组形式存在于方法中 System.out.println(Arrays.toString(args)); }*/ public <T> void add(T...args) { System.out.println(Arrays.toString(args)); } |
public interface MyInterface<T> { public void showInfo(T a); } |
public class ImplClass implements MyInterface<String>{ @Override public void showInfo(String a) { // TODO Auto-generated method stub } } |
public class ImplClass2<T> implements MyInterface<T>{ @Override public void showInfo(T a) { // TODO Auto-generated method stub } } |
public static void print(ArrayList<? extends Pet> list) { for(Pet pet:list) { pet.showInfo(); } } |
public static void main(String[] args) { ArrayList<String> list = new ArrayList<String>(); list.add("apple"); list.add("banana"); list.add("coco"); // 需求:在遍历的过程中,添加元素 Iterator<String> it = list.iterator(); while(it.hasNext()) { String item = it.next(); if(item.equals("banana")) { // list.add("test"); } } System.out.println(list); ListIterator<String> it2 = list.listIterator(); while(it2.hasNext()) { String item = it2.next(); if(item.equals("banana")) { it2.add("test"); } } System.out.println(list); } |
Exception in thread "main" java.util.ConcurrentModificationException at java.util.ArrayList$Itr.checkForComodification(ArrayList.java:909) at java.util.ArrayList$Itr.next(ArrayList.java:859) at cn.sxt06.iterator.Test01.main(Test01.java:18) |
public static void main(String[] args) { /** * 增:add/addAll * 删:clear/remove/removeAll/retainAll * 改: * 查:contains/containsAll/isEmpty/equals/size * 遍历:iterator */ Set<String> set = new HashSet<String>(); // 【1】添加 boolean r; r = set.add("apple"); System.out.println(r); set.add("coco"); set.add("banana"); r = set.add("apple"); // 没有添加成功 System.out.println(r); // 【2】删除 // set.clear(); set.remove("coco"); System.out.println(set); } |
public static void main(String[] args) { Set<String> set = new HashSet<String>(); set.add("apple"); set.add("coco"); set.add("banana"); // foreach for(String str:set) { System.out.println(str); } Iterator<String> it = set.iterator(); while(it.hasNext()) { System.out.println(it.next()); } } |
![]() |
package cn.sxt02.hashset; public class Student { private String id; private String name; private int age; // … @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + age; result = prime * result + ((id == null) ? 0 : id.hashCode()); result = prime * result + ((name == null) ? 0 : name.hashCode()); return result; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; Student other = (Student) obj; if (age != other.age) return false; if (id == null) { if (other.id != null) return false; } else if (!id.equals(other.id)) return false; if (name == null) { if (other.name != null) return false; } else if (!name.equals(other.name)) return false; return true; } @Override public String toString() { return "Student [id=" + id + ", name=" + name + ", age=" + age + "]"; } } |
![]() |
TreeSet<Integer> set = new TreeSet<Integer>(); set.add(2); set.add(3); set.add(1); set.add(4); set.add(3); System.out.println(set); |
package cn.sxt04.treeset; public class Student implements Comparable<Student>{ private String id; private String name; private int age; // . . . @Override public int compareTo(Student o) { // 按照年龄比较 if(this.getAge() < o.getAge()) { return -1; }else if(this.getAge() == o.getAge()) { return 0; }else { return 1; } } } |
@Override public int compareTo(Student o) { // return this.getAge() - o.getAge(); // 按照年龄比较 if(this.getAge() < o.getAge()) { return -1; }else if(this.getAge() == o.getAge()) { /*if(this.getName().compareTo(o.getName()) < 0) { return -1; }else if(this.getName().compareTo(o.getName()) == 0) { return 0; }else { return 1; }*/ return this.getName().compareTo(o.getName()); }else { return 1; } } |
public class Test04 { public static void main(String[] args) { LenCompare lenCompare = new LenCompare(); TreeSet<String> set = new TreeSet<String>(lenCompare); set.add("alex"); set.add("ben"); set.add("cocos"); set.add("scott"); // 需求:按照字符串的长度升序? System.out.println(set); } } class LenCompare implements Comparator<String> { @Override public int compare(String o1, String o2) { // [1] 按名称长度升序 // System.out.println("o1:"+o1); // System.out.println("o2:"+o2); // return o1.length() - o2.length(); // [2] 先按照名称长度,如果相等,按名称自然顺序 /*if (o1.length() == o2.length()) { return o1.compareTo(o2); } return o1.length() - o2.length();*/ // [3]按照长度降序 return o2.length() - o1.length(); } } |
package cn.sxt04.treeset; import java.util.Comparator; import java.util.TreeSet; public class Test05 { public static void main(String[] args) { LenCompare lenCompare = new LenCompare(); TreeSet<String> set = new TreeSet<String>(new Comparator<String>() { @Override public int compare(String o1, String o2) { return o1.length() - o2.length(); } }); set.add("alex"); set.add("ben"); set.add("cocos"); set.add("scott"); // 需求:按照字符串的长度升序? System.out.println(set); } } |
![]() |
package cn.sxt05.map; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; public class Test01 { public static void main(String[] args) { /** * 增:put/putAll * 删:clear/remove * 改:put * 查:get/containskey/containsValue/isEmpty/size/values * 遍历:entrySet/keySet */ Map<String, ArrayList<String>> map = new HashMap<String,ArrayList<String>>(); // 【1】添加 ArrayList<String> list1 = new ArrayList<String>(); list1.add("alex"); list1.add("alice"); list1.add("allen"); map.put("A", list1); ArrayList<String> list2 = new ArrayList<String>(); list2.add("ben"); list2.add("bill"); map.put("B", list2); ArrayList<String> list3 = new ArrayList<String>(); list3.add("coco"); list3.add("cill"); map.put("C",list3); System.out.println(map); // 【2】删除 // map.clear(); // map.remove("C"); // System.out.println(map); // 【3】修改 ArrayList<String> list4 = new ArrayList<String>(); list4.add("doco"); list4.add("dill"); // 更新 map.put("C",list4); System.out.println(map); // 【4】获取 ArrayList<String> list = map.get("C"); System.out.println(list); // 其他 // System.out.println(map.containsKey("C")); ArrayList<String> list5 = new ArrayList<String>(); list5.add("doco"); list5.add("dill"); System.out.println(map.containsValue(list5)); System.out.println(map.size()); // 获取所有的value到一个collection中 System.out.println(map.values()); } } |
package cn.sxt05.map; import java.util.ArrayList; import java.util.HashMap; import java.util.Iterator; import java.util.List; import java.util.Map; import java.util.Map.Entry; import java.util.Set; public class Test02 { public static void main(String[] args) { /** * 遍历:entrySet/keySet */ Map<String, String> map = new HashMap<String, String>(); map.put("B", "banana"); map.put("C", "coco"); map.put("A", "apple"); // map是无序的。如果想要map有序,选择key是一定要具有可排序性。一般建议用string作为key // 【1】获取所有的key Set<String> keySet = map.keySet(); Iterator<String> it = keySet.iterator(); while (it.hasNext()) { String key = it.next(); System.out.println(key + "=>" + map.get(key)); } // 快速遍历forearch??? // 【2】获取所有的entry Set<Entry<String, String>> entrySet = map.entrySet(); Iterator<Entry<String, String>> it2 = entrySet.iterator(); while(it2.hasNext()) { Entry<String, String> entry = it2.next(); System.out.println(entry.getKey()+"=>"+entry.getValue()); } // 快速遍历forearch??? System.out.println(map); } } |
public class Test01 { public static void main(String[] args) { HashMap<Student, String> map = new HashMap<Student,String>(); Student s1 = new Student("001", "大狗", 20); Student s2 = new Student("002", "2狗", 21); Student s3 = new Student("003", "3狗", 22); Student s4 = new Student("002", "2狗", 21); map.put(s1, "apple"); map.put(s2, "banana"); map.put(s3, "coco"); map.put(s4, "bill"); System.out.println(map); } } |
package cn.sxt07.linkedhashmap; import java.util.LinkedHashMap; public class Test01 { public static void main(String[] args) { LinkedHashMap<Student, String> map = new LinkedHashMap<Student,String>(); Student s1 = new Student("001", "大狗", 20); Student s2 = new Student("002", "2狗", 21); Student s3 = new Student("003", "3狗", 22); Student s4 = new Student("002", "2狗", 21); map.put(s3, "coco"); map.put(s1, "apple"); map.put(s2, "banana"); map.put(s4, "bill"); System.out.println(map); } } |
package cn.sxt08.treemap; import java.util.TreeMap; public class Test02 { public static void main(String[] args) { TreeMap<Student, String> map = new TreeMap<Student,String>(); Student s1 = new Student("001", "大狗", 20); Student s2 = new Student("002", "2狗", 21); Student s3 = new Student("003", "3狗", 22); Student s4 = new Student("002", "4狗", 21); map.put(s3, "coco"); map.put(s1, "apple"); map.put(s2, "banana"); map.put(s4, "bill"); System.out.println(map); } } |
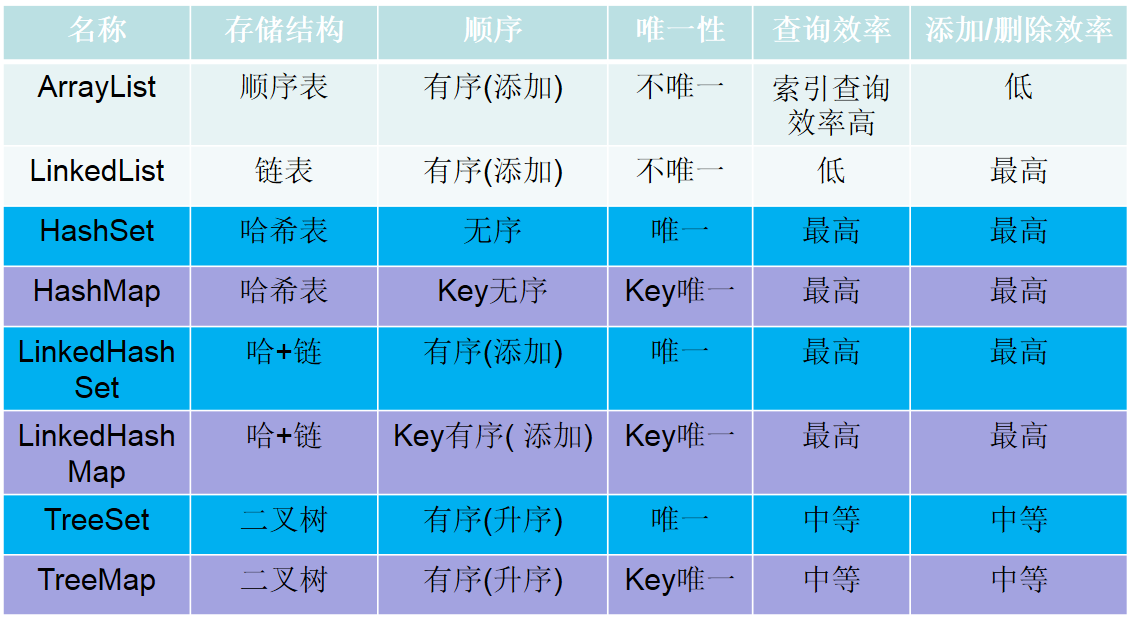
相关文章
- 暂无相关文章
用户点评